A common requirement when working with item collection controls such as list box is validation.The user should only be able to submit the form when he has selected some values in the listbox.
We can easily implement this scenario on the client side using javascript and jQuery.For example if we define the select element as:
<select id="products" multiple > <option value="1" selected>Item 1</option> <option value="2">Item 2</option> <option value="3">Item 3</option> <option value="4">Item 4</option> <option value="5">Item 5</option> </select>
The above listbox is rendered as:
Client side validation using javascript
To allow the user to submit the form only when some value is selected in the listbox we can implement a javascript validation function.The function will validate if any item is selected in the listbox.If no item is selected then it will not allow the user to submit the form
<select id="products" multiple > <option value="1" selected>Item 0</option> <option value="2">Item 1</option> <option value="3">Item 2</option> <option value="4">Item 3</option> <option value="5">Item 4</option> </select> <input id="submit" type="submit" value="submit" onclick="return Validate();" />
The javascript function is defined as
function Validate() { var ddl = document.getElementById("products"); if (ddl.selectedIndex==-1) return false; else return true; }
Client side validation using jQuery
We can write the above validation function using jQuery as:
function Validate() { var noOfSelectedItems=$('#products :selected').length; if (noOfSelectedItems==0) return false; else return true; }
Instead of preventing the postback we can also completely disable the submit button if no items are selected in the listbox
function Validate() { var ddl = document.getElementById("products"); var noOfSelectedItems=$('#products :selected').length; if (noOfSelectedItems == 0) { $("#submit").prop("disabled", true); } else { $("#submit").removeAttr('disabled'); } }
We can call the Validate() function whenever an item in the listbox is selected:
<select id="products" multiple onchange="Validate()"> <option value="1" selected>Item 0</option> <option value="2">Item 1</option> <option value="3">Item 2</option> <option value="4">Item 3</option> <option value="5">Item 4</option> </select>

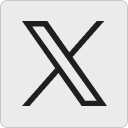
Leave a Reply