When developing views in MVC we use html helper methods for creating different user interface
elements such as the textbox and radio buttons.There are different html helper methods
for creating UI elements.Most of these html helper methods have
two versions strongly typed which uses lambda expressions and the one which takes string value as a parameter. For more details please refer HTML Helper methods
Using most of these helper methods is similar as we need to pass the name
and the value which we want to render in the generated HTML.Usage of DropDownList helper method is different since a Dropdown can have multiple elements which the user can select. And for each item in dropdown both value and text properties needs to be set.
Dropdown is rendered as:
<select name="itemsList"> <option>Item1</option> <option>Item2</option> <option>Item3</option> <option>Item4</option> <option>Item4</option> <option>2015</option> </select>
To populate dropdownlist in mvc we need to do the following:
- In the view we need to call the Html.DropDownList() method with the proper arguments.Below is one overload of the method which we can use
Html.DropDownList(“itemsList”)
What above method will do is that it will look in the viewdata or viewbag for a property with the name itemsList and once it finds that property it will render the SelectListItems in the viewdata. - In the action method we need to populate the property of the viewdata which we are passing to the Html.DropDownList() method in the view
For populating the property of the viewdata in the controller: - 1.Create a list of SelectListItem.Assign proper values to the value and text properties of the SelectListItems as these will be used while rendering the dropdownlist
- 2.Assign these values to the viewdata property.
In the following example we are assigning some string values to a List collection of type SelectListItem.Also we are assigning the items collection to the itemsList key in ViewData
public ActionResult Index() { List<SelectListItem> items = new List<SelectListItem>(); items.Add((new SelectListItem { Text = "C#", Value = "1" }); items.Add(new SelectListItem { Text = "Java", Value = "2" }); items.Add(new SelectListItem { Text = "Php", Value = "3" }); items.Add(new SelectListItem { Text = "SQL", Value = "4" }); ViewData["itemsList"] = items; return View(); }
The html helper searches the itemsList key in the ViewData and renders the values it finds in the collection using the text and value properties we have assigned to the SelectListItem to render the options in the select html
Above SelectListItems will render the following dropdown

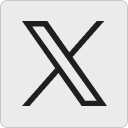
Leave a Reply