with statement was introduced in Python 2.5 and is quite useful when you want to work with a resource and then want to dispose/close it when you are done.
The with statement is used for writing blocks of code when we want to call methods as defined by context.It allows to write block of code in a more concise way then
try finally block.The with statement is used to wrap the execution of a block with methods defined by a context manager
you use with statement as:
specify expression after with statement followed by target variable
with expression as variable
when the above statement is executed the __enter__ method of the expression result is called and the result is assigned to the given variable.
In following example we are creating an object of file using the with statement.The benefit of using with statement here is that the file object will be automatically closed after the block executes.Even if exception occurs in the while block ,the file will still be closed.
import csv with open('csvSample.csv') as file: csv_values = csv.DictReader(file) for row in csv_values: print("%s,%s"%(row['column1'],row['column2']))
Without using with statement we will have to close the file explicitly.Same logic as the above sample can be written without using with statement as:
file = open('csvSample.csv') csv_values = csv.DictReader(file) for row in csv_values: print("%s,%s"%(row['column1'],row['column2'])) file.close()
The above logic is not as concise as the previous one.Not only this if an exception happens file object will not be closed unlike the with statement.When we use with statement the file is guaranteed to be closed whether exception happens or not.
If you want to use your custom class in with statement then you need to define the enter and exit methods:
class SampleClass(): def __init__(self, value): # constructor logic def __enter__(self): # create logic def __exit__(self, type, value, traceback): #destroy logic
The above is just a pseudo code,but you can use it to define actual class which can be used in with statement.

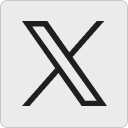
Leave a Reply