When a c# application is compiled an assembly is generated .This assembly contains the compiled source code and the metadata.The source code in the assembly is in the form Intermediate Language or IL.The metadata that the assembly contains is required in different scenarios like investigating an unknown assembly at runtime .We can query the metadata from the assembly using reflection.
Some of the common uses of reflection are
- Using reflection we can view the types in an assembly at runtime.We can also instantiate these types.
- We can get type attribute information at runtime
- We can investigating an unknown assembly at runtime
To use reflection in c# we need to use the reflection api and the Type class.The reflection api is defined in the System.Reflection namespace and the Type class is defined in the System.Type namespace.One of the most important types defined in the reflection api is MemberInfo.It is an abstract class and several subclasses derive from it.These classes provides information about different program elements.
[table id=3 /]
We can obtain a type object using the typeof() operator.To obtain a type object of string type we can use the following
Type typeObj=typeof(string);
Once we get the type object we can retrieve information about it using the different methods. In the below example we are retrieving the information about a string method at runtime.
static void Main(string[] args) { Type typeObj=typeof(string); Type[] paramTypes = {typeof(int)}; MethodInfo mi = typeObj.GetMethod("Substring", paramTypes); Console.WriteLine("Name={0},Return Type={1},IsAbstract={2}",mi.Name,mi.ReturnType,mi.IsAbstract); Console.ReadLine(); }
If we execute the above code we get some of the details about the string method.
In the above code we are using the GetMethod() of the type class which returns the information about the method defined by the string class.
Some of the important methods defined by the Type class are
[table id=2 /]
To get the constructors defined by the string class we can use the below code
Type typeObj = typeof(string); ConstructorInfo[] p = typeObj.GetConstructors(); Console.WriteLine(p.Length); for (int i = 0; i < p.Length; i++) { Console.WriteLine(p[i].ToString()); } Console.ReadLine();
On executing the above code we get the signatures of the different constructors defined by the string class.

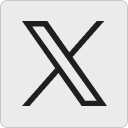
Leave a Reply