TypeScript provides man useful features which supports maintenance and better code management.One such feature which is available in object oriented languages such as c# is the concept of classes.We can declare classes in TypeScript.
Following a simple class in TypeScript which contains a single method and a field.The TestClass class declares a field called firstName.firstName is declared as a string field using type annotations.We can mark variables and method parameters with type annotations which specifies the type of variable or method parameter.
TestClass class also declares a constructor which accepts a single parameter of type string.
class TestClass{ firstName:string; constructor (name: string) { this.name = firstName; } GetName() { return firstName; } }
So the two important TypeScript concepts we can see from the class declared above are:
- Type annotations describe the data type of a variable or a method argument
- constructor are declared using the keyword constructor.
- Fields in a class are accessed using the this keyword.
We have modified the above program to display the firstName as:
class TestClass { firstName: string; constructor(name: string) { this.firstName = name; } GetName() { return this.firstName; } DisplayName() { alert('Hello ' + this.firstName); } } window.onload = () => { var obj = new TestClass("User1"); obj.DisplayName(); };
When we compile the above class the following javascript class is generated.The javascript code simulates the class in TypeScript through the javascript conceptsĀ of closures and prototypes.TestClass class is converted to a self invoking function.
var TestClass = (function () { function TestClass(name) { alert('aaaaaaaaaaa'); this.firstName = name; } TestClass.prototype.GetName = function () { return this.firstName; }; return TestClass; }());

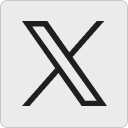
Leave a Reply