Generics is concept used in different programming languages.Generic are used for defining reusable elements such as classes,methods and interfaces.
In TypeScript you can define Generic Classes,Interfaces and functions.Generic functions ,generic interfaces and generic classes work with different data types using the concept of type parameters.
Type parameter is a parameter which specifies the data type of a generic Class or Interface.When we create an object a generic type we specify type parameter.The specified type parameter determines the type of the generic type.
Generic parameters are defined in a different manner then normal parameters.Normal method parameters are defined as comma separated values inside paranthesis “(” and “)”.Generic parameters are instead specified inside angle brackets “<” and “>”.For example you can specify a type parameter called T as <T>.T represents the type used by the generic class or interface.
Type parameter are part of the type.For example you can define a generic collection object as:
var collObj= new Collections.Set<number>();
Generic Class
Generic class uses type parameters to define a class which will work with different data types.For example in the following generic class T is the type parameter.
class Department<T> { //different types of employees private employees:Array<T>=new Array<T>(); add(employee:T) { this.employees.push(employee); } }
You can define a class which will work with HREmployee type as:
let hrDepartment:Department<HREmployee>=new Department<HREmployee>();
Generic function
Generic function works with different data types by defining generic parameters.In the following example we have declared a Generic function called GenericFunction. The type passed to this function is called T.We are using T to declare the type
of parameters passed to the GenericFunction function.We are also using T to declare the return type of the function.
function GenericFunction<T>(x:T):T { return x*x; }
You can call the GenericFunction by using the following syntax:
GenericFunction<int>(1);
Generic Interface
You define a generic interface by using the keyword interface followed by the interface name and the the type parameters.Following interface declares a single method which will return value of type parameter T.
interface GenericInterface<T>{ getEmployee:()=>T; }
You can use generic interface to implement a generic class as:
class GenericClass<T> implements<T>{ }

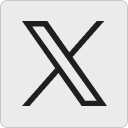
Leave a Reply