There are few different conditional statements in TypeScript such as If ..else.One useful conditional statement is switch..case.
The switch statement is used to test the value of an expression against multiple constant values.We provide the expression as input to the switch statement.The switch statement contains multiple case statements.The expression is compared against the label constant of each case.When the expression matches the constant then the statements in that case are executed
If no case matches the expression then the statements of the default case are executed.This flow can be depicted using the
following diagram:
Following is an example demonstrating the use of switch statement in TypeScript.We have declared a class called Products.In this class we have declared a function called findItem.We are passing a parameter to the findItem() function.
The findItem() function declares a switch statement.Based on the matching expression value appropriate message is displayed.
class Products{ element: HTMLElement; div: HTMLElement; constructor(element: HTMLElement) { this.element = element; this.div = document.createElement('div'); this.element.appendChild(this.div); } findItem(item: string) { switch (item) { case "laptop": { this.div.innerHTML = "laptop found"; alert("laptop"); break; } case "desktop": { alert("desktop"); this.div.innerHTML = "desktop found"; break; } default: { this.div.innerHTML = "item not found"; alert("not found"); break; } } } } window.onload = () => { var el = document.getElementById('items'); var products= new Products(el); products.findItem("mp3"); };
Fall through Case
Following is an example of fallthrough case statement
case 0: statements; case 1: statements; break;
If we turn on the following switch then the above statement is invalid in TypeScript
–noFallthroughCasesInSwitch
However the following statement is valid since thefirst case is empty
case 0: case 1: statements; break;

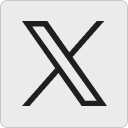
Leave a Reply