This tutorial will help you understand the basics of TypeScript if you don’t have any experience with TypeScript.
TypeScript
TypeScript is a language which is used for building large JavaScript applications.JavaScript is not suitable for developing large scale enterprise applications like other programming languages,such as Java or C#.
TypeScript helps in developing large JavaScript applications in the following ways.
It adds language features which were missing in JavaScript and which are required for developing large scale applications.These features are:
Object Oriented language features such as:
- Classes
- Interfaces
- Inheritance
Optional Static typing.TypeScript is a strongly typed language.This means that the type checking is performed at compile time instead of run time.
Compilation.TypeScript is a compiled language.JavaScript is an interpreted language.This means that type errors are caught at compile time instead of runtime unlike JavaScript.
Intellisense support
Main features of TypeScript can be depicted with the following diagram:
For understanding what is TypeScript Overview of TypeScript
Creating a simple application using TypeScript
For developing TypeScript applications we can use:
- Visual Studio 2013(update 2) and Visual Studio 2015
- Visual Studio Code
- Any Text Editor
If you are using text editor you can install TypeScript using the following Node.js npm command:
npm install -g typescript
Since TypeScript is a superset of JavaScript so a JavaScript program is also a valid TypeScript program.Following is a simple JavaScript program which is also a valid TypeScript program:
function Hello(message) { document.body.innerHTML = message; } Hello("Hello TypeScript");
Save above code in a file Hello.ts.TypeScript files have .ts extension by convention.
Compiling a TypeScript program
If you are using text editor you can compile the above code using the TypeScript transpiler as:
tsc greeter.ts
Classes in TypeScript
A real world TypeScript application consists of classes and interfaces.To understand the basics of classes in TypeScript :Class in TypeScript
Classes in TypeScript are similar to classes in Java and C#.Classes are declared using the keyword class.Following declares an Employee class:
class Employee{ constructor(public Name) { this.fullName =Name; } }
Important point to consider in the above code is Name argument is declared as public.When a constructor argument as declared as public in TypeScript then property with the same name is automatically created by TypeScript.Here Name property is created automatically.
Type Annotations
Annotations are used declare variables and function parameters in TypeScript.We declare a variable using annotation as:
varName:TypeName;
We declare a string variable as:
firstName: string;
In function arguments we use annotations as:
function Hello(message:string) { document.body.innerHTML = message; }
Variable declaration in TypeScript
Variable Declaration in TypeScript
Arrays in TypeScript
Interface in TypeScript
Interface in TypeScript is similar to interface in Java and C#.Interface is declared using interface keyword.
interface CommonInterface { Name: string; Address: string; }
An important feature of interfaces in TypeScript is that interfaces in TypeScript doesn’t need to be implemented explicitly.So the following class implicitly implements the above interface.This is because in TypeScript if the structure of a class is similar to structure of interface then it implicitly implements it.
class Employee { constructor(public Name, public Address) { } }

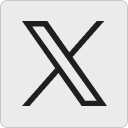
Great docs .