We can define routes in asp.net core in many ways.The two most common approaches for defining routes in asp.net core:
- Conventional routing
- Attribute-based routing
Conventional routing
Conventional routing is the traditional approach of routing.In this approach routes are set up during application launch in the StartUp class’s method
In this approach you define application routes while calling the AddMvc method.You pass the routes as a parameter to this method.To do this you use the MapRoute() method.
Below is the default route created by dot net core when you create a new application
app.UseMvc(routes => { routes.MapRoute( name: "default", template: "{controller=Home}/{action=Index}/{id?}"); });
Here we are using the following parameters of the MapRoute method:
name name of the route,every route should be given some name
template this is the url structure that will map the url with the action method.This can contain tokens which are enclosed in curly braces.For example {action} ,{controller} anf {id?} are tokens.
this route will map the request such as www.electronicitems.com/Items/Home to Items action in the home controller.Since we have assigned the default values if we call the www.electronicitems.com then it will map to the Index action in Home controller.Also id is optional ,if you don’t pass id then your application will not break.
Attribute-based routing
This uses attributes for defining routes.Two of the attributes used for implementing attribute based routing are:
- Route
- Http +HTTP Verb+Attribute[(url template)]
[Route(“api/Employees”)]
If we add this attribute to the controller then the request for the url begining with api/Employees will match this controller.
Other approach to implement attribute based routing in your code is to use HttpGet,HttpPost or other attribute corresponding to the Http verb.
For example to match the url api/Employees to the below method we can define the following route.This will also match the url api/Employees
[HttpGet("it")] public string[] GetEmployees() { //statements }

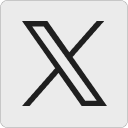