RecyclerView is successor to ListView.It is more efficient in displaying large datasets without too much overhead.RecyclerView consists of two main components to achieve this:
- adapter
- layout manager
To use RecyclerView you need to add the RecyclerView in the view of the activity
In the activity class retreivethe RecyclerView using the findViewById() method
Set the adapter using the setAdapter() method
recyclerView.setAdapter(adapter);
Set the Layout manager using the setLayoutManager() method
recyclerView.setLayoutManager(layoutManager);
RecyclerView has these builtin layout managers:
- LinearLayoutManager
- GridLayoutManager
- StaggeredGridLayoutManager
<RelativeLayout xmlns:android=”http://schemas.android.com/apk/res/android”
xmlns:tools=”http://schemas.android.com/tools”
android:layout_width=”match_parent”
android:layout_height=”match_parent”
tools:context=”com.androidcookbook.recyclerviewdemo.ListActivity”>
<android.support.v7.widget.RecyclerView
android:id=”@+id/recyclerView”
android:layout_width=”match_parent”
android:layout_height=”match_parent”/>
</RelativeLayout>
An important concept in Adapter of RecyclerView is ViewHolder.List items contains reference to ViewHolder objects.ViewHolder implementation should cache the expensive findViewById(int) methods.

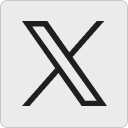