ListView is used to display multiple items at a time.The items are displayed in rows.This allows the user to view multiple items at a time.Also the user can select the items from the list view.
To use listview in a android application you first define the layout or view of the Activity.In this layout you declare the ListView.Data is provided to the ListView using the setListAdapter() method.There are several implementations of the ListAdapter.But the most basic is BaseAdapter in the android.widget package.Rows are created by the adapter
To use ListView in Android application first declare the <ListView> XML element in the layout.
<ListView android:id="@+id/ListView01" android:layout_width="wrap_content" android:layout_height="fill_parent"/>
In the Activity class retreive the ListView by calling the findViewById() method.
ListView listView = (ListView) findViewById(R.id.ListView01);
Create a ListAdater as:
BaseAdapter adapter=new BaseAdapter() { public int getCount() { return words.length; } public Object getItem(int position) { return words[position]; } public long getItemId(int position) { return position; } public View getView(int position, View convertView, ViewGroup parent) { LayoutInflater inflater = (LayoutInflater) getSystemService(LAYOUT_INFLATER_SERVICE); View view = inflater.inflate(R.layout.list_row, null); TextView textView = (TextView) view.findViewById(R.id.TextView01); textView.setText(words[position]); return view; }
Set the ListAdapter by calling the listView.setAdapter() method and passing the ListAdapter created in the above step
listView.setAdapter()
Define a layout for every row in the ListView in the res/layout directory:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="wrap_content" android:layout_height="wrap_content"> <TextView android:text="@+id/TextView01" android:id="@+id/TextView01" android:layout_width="fill_parent" android:layout_height="wrap_content"/> </LinearLayout>

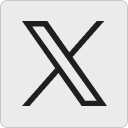
Leave a Reply