Saving or persisting data is required any application.There are different ways available for persisting data in Android App.
- Internal storage is the storage that is available in the device without any extension card.This is also called flash memory.
- External storage is present in the removable devices.
- Shared Preference for storing application specific custom values in key,value pairs.
- SQLite for storing large amounts of data in RDBMS.
Internal storage
For reading the files stored in the internal storage you use the java file apis.
For reading the file:
Context methods openFileInput(String filename), which returns a FileInputStream object
For writing to the file:
openFileOutput(String filename, int mode), which returns a FileOutputStream.
External storage
The Environment class provides few type which can be used for working with external storage.Such as the DIRECTORY_MUSIC for playable music
Shared Preferences
To store application specific values you use shared preferences.
To put values in shared preferences you obtain reference to sharedpreferences object as:
sharedpreferences = getSharedPreferences(MyPREFERENCES, Context.MODE_PRIVATE);
After this you put the values in sharedprefernce as key value pairs as:
sharedpreferences = getSharedPreferences(MyPREFERENCES, Context.MODE_PRIVATE); sharedPreferences.edit().putBoolean("booleanValue", true).commit(); sharedPreferences.edit().putString("sringValue","sampleString")
SQLite
SQLite is an Open Source database. SQLite supports standard relational database features like SQL syntax, transactions and prepared statements. The database requires limited memory at run time (approx. 250 K Byte) which makes it a good candidate from being embedded into other run times.
SQLite is database used in mostly mobile and other embedded devices since doesn’t require any setup and configuration on the device.Other advantage that makes it a good database for embedded devices is that it has low memory footprint ,about 250 KByte.
To implement SQLite data access in your application you use the SQLiteOpenHelper class.You implement a subclass of SQLiteOpenHelper class.In this subclass you override the following methods:
- onCreate
- onUpgrad

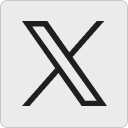
Leave a Reply