Change detection is the mechanism which is used to propagate changes in the model to the view.Change detection mechanism in Angular 2 is much better than the previous version.
Data binding in AngularJS depended on the digest cycle.Whenever there is a change in any of the source or destinations involved in a binding all of the bindings in the application are reevaluated.Digest cycle consists of watchers whose responsibility is to watch for the changes in any of the expressions.These watchers are
evaluated multiple times for a change in even single expression.So you can imagine the performance overhead of the digest cycle.
Since you have changed only a single expression it doesn’t make much sense to reevaluate all the expressions in the application.
Angular 2 in contrast to previous versions of Angular has a much better change detection strategey. Every Angular 2 component has a dedicated
change detector.
In the following component we have declared onChange() method.This method will be called whenever use clicks a button.
This will create change detector whose job is to check if the value of the expression has changed.Here it checks if the value of name property has changed.
@Component({
selector: 'example-component',
template: '<button (click)="onChange()">{{name}}</button>'
})
export class TodoItem {
public name:string="Angular 1.0";
onChange() {
name:string="Angular 2.0";
}
}
The default change detection mechanism in Angular 2.0 is 3 to 10 times faster than Angular 1.0.But thats not all.We can even tweak the default change detection mechanism.This is made possible through the ChangeDetectionStrategy API.The ChangeDetectionStrategy API defines when the change detection should be triggered.
Some of the useful attributes of this API are:
- Detached Detached means that the change detector sub tree is not a part of the main tree and should be skipped.
- OnPush OnPush means that the value of the component can change only through user inputs and events
We can update the previous component by setting the changeDetection attribute to ChangeDetectionStrategy.OnPush
@Component({
changeDetection: ChangeDetectionStrategy.OnPush,
selector: 'example-component',
template: '<button (click)="onChange()">{{name}}</button>'
})
export class TodoItem {
public name:string="Angular 1.0";
onChange() {
name:string="Angular 2.0";
}
}
What the above component will do now is it will update view only in specific scenarios.
- When some event is fired.
- When value of input changes.

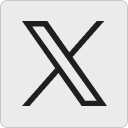
Leave a Reply