Dependency injection is useful for developing decoupled applications.Dependency Injection in Angular 2 is handled by the framework.
Dependency Injection in Angular 2 Components
In angular 2 to specify the dependencies of a component ,just specify the dependencies as the constructor parameters.Just adding the dependencies as constructor parameters informs the angular 2 framework that the component needs some dependencies to be injected.
@Component({ selector: 'org-comp', }) export class OrganizationComponent {Department constructor(Department: DepartmentService) { }
In the above code we have specified that our OrganizationComponent depends on the DepartmentComponent. Angular 2 framework will create an instance of DepartmentComponent and inject it into the constructor.
We have declared DepartmentComponent as:
@Component({ selector: 'emp-comp', }) export class DepartmentComponent { constructor(Employee: EmployeeComponent) { }
DepartmentComponent Depends on the EmployeeComponent so Angular framework will create an instance of EmployeeComponent when it tries to create an instance of DepartmentComponent. Best thing about this is that you don’t need to be bothered about this hierarchy of dependencies since it is automatically taken care of
by angular framework.
Dependency injection in Services using @Injectable()
Since we have used components to inject dependencies above so we don’t need to do any other thing than to declare dependencies as constructor parameters.But if we use service then to specify that a service has some dependencies you need to decorate the service with the @Injectable() decorator.
@Injectable() export class EmployeeDetailsService { constructor(private detailsService: DetailsService) { } }
In the above service implementation of EmployeeDetailsService you can notice that it has a dependency on DetailsService service.Since EmployeeDetailsService depends on DetailsService so we have used the @Injectable() decorator function.

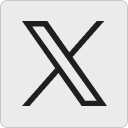
Leave a Reply