URLs identify resources which the user can request from the server.For example to request products from a website user could append the following to the domain of the website.
/products/list
But this url might also contain specific details which user wants to request such as the product id.User can pass these details in the url as:
/products/list/id
this is where route parameters are used in Angular.When implementing routing in application, route parameter allows user to pass details about the resource which he is requesting.Based on these details angular can load the corresponding component.
To implement parameterized routes in your application you need to first declare routes in your application.
Define routes in the app module
In the app module(where we usually define application routes) pass parameters to the RouterModule.forRoot() method .In the following route we are defining id as a parameter ,this route will be handled by the categoryComponent component.
RouterModule.forRoot([ { path: 'category/:id/:subid', component: categoryComponent } ])
Using RouterLink for declaring routing links
In the view you need to define the anchor tag which will pass the parameters you have defined in the router.You add such routing functionality to the view using RouterLink directive in the view.
In Angular “routerLink” is a directive which allows user to navigate between different routes.You pass the the pattern to match to the routerlink directive.For example in the following routerlink directive we are passing multiple parameters-
<a [routerLink]="['/category','CategoryA','productA']"> product A </a>
Passing route parameters
You can pass router parameters by using the queryParams directive as:
<a [routerLink]="['../']" [queryParams]="{propertyName: 'value'}">Main Link</a>
In the constructor of the above component(categoryComponent) inject “ActivatedRoute” as a dependency.You would also need to import the @angular/router module as:
import { ActivatedRoute, Router } from '@angular/router';
Inject ActivatedRoute as a dependency in constructor
constructor( private activeRoute: ActivatedRoute ){ }
Fetch route parameters in component
In the ngOnInit fetch parameter in component
ngOnInit() implements OnInit{ this.activeRoute.queryParams.subscribe(params => { let id = params['id']; let subid = params['subid']; FetchDataService(id,subid); }); }
usually you pass these parameters to any service for fetching the data
public id:string=""; public data:string[]=[]; constructor( private activedRoute: ActivatedRoute, private service: DataService) { this.activedRoute.params.subscribe(params=> { console.log('in subscribe'); this.id=params["id"]; service.get(this.id).subscribe((response)=> { this.data=response; } } }
navigateByUrl
This method is defined by the router service and is used for performing navigation through code.To use this method you need to first pass the router service as a dependency.
constructor(private router:Router){ }
You can then use “navigateByUrl” method in the code for navigating to a matched pattern.You pass the URL of the view to navigate to as an argument to the navigateByUrl method.You pass this URL as a string.
You can call method in your code from the html template and then navigate to a different route using this method.
onClick(){ this.router.navigateByUrl('/category/1'); return true; }
navigate
This is similar to the navigateByUrl method except this method accepts array of URL segments and not string.

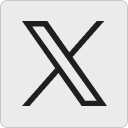
Leave a Reply