AngularJS framework is based on the Model View Controller architectural pattern for creating Rich Internet Applications.Controller is an important component of any AngularJS application.Controller in AngularJS acts as a glue between the model and the view and has the following important responsibilities:
• Define the model object which can be accessed from the view
• Define the business logic of our application
View is a template whose responsibility is just rendering the model using the data bindings.The model object state and behaviour is defined by the controller.
Controller is defined using a javascript constructor function.This function is passed a $scope object.We set the state of the $scope object inside the controller by setting values on the $scope object.These property values on the $scope object represent our Model which is accessible from the view template.
We attach the controller to the DOM element using the ng-controller directive.Setting the ng-controller directive on a DOM element creates a new controller object using the controller constructor function.We can then access the $scope object properties from the view.
Though we can define the controller function directly but defining it as part of a module helps to keep the code organized.In the following example we are creating a controller as part of a module and then attaching it to a view.
1.Create a module
First we need to create a Angular module using the angular object.We will name our module myApp
var myApp = angular.module('myApp',[]);
2. Create controller using the module object
Once we have a module object we create a controller using the controller() function of the angular module.We need to assign this controller to the view using the ng-controller directive
myApp.controller('TestController', ['$scope', function($scope) { //method body }]);
We are passing the second argument [‘$scope’ to indicate that our controller function depends on the $scope object
3. Set state on the $scope object inside the controller
$scope is the object to which we assign the model object. We assign the Message property to the $scope object.$scope object is passed by the framework to the controller function.
myApp.controller('TestController', ['$scope', function($scope) { $scope.Message= 'Hello world!'; }]);
4.Attach the view to the controller
To associate our view to the controller we assign the value TestController to the ng-controller directive of the div.We also set the ng-app directive as our module on this div ,this will limit the scope of our angular application to the div.
<div ng-app="myApp" ng-controller="TestController"> {{Message()}} </div>
Inside the div we are accessing the $scope.Message property using a javascript expression.
Defining Behaviour
myApp.controller('TestController', ['$scope', function($scope) { $scope.Message= 'Hello world!'; $scope.SetDate = function() { var date = new Date(); return date.toUTCString(); } }]);
<div ng-app="myApp" ng-controller="TestController"> Date {{SetDate()}} </div>
Above we are calling the SetDate() using a javascript expression.Usually we need to call a function on button click event,we can call a function defined on the $scope object using ng-click directive.
<button ng-click="SetDate()">SetDate</button> <div>{{Date}}</div>
In the above example we are calling the SetDate() method on a button click.Instead of returning a date from the controller function we are now assigning it to the Date property.Using $scope object is the proper way to set state instead of directly calling a function from the view.
$scope.SetDate = function() { var date = new Date(); $scope.Date=date.toUTCString(); }

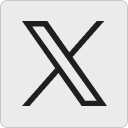
Good articles, i would recommend to you: https://github.com/johnpapa/angularjs-styleguide , for better readability and avoiding memory leaks.
Sincerely,
Robbert
Thanks! .I am glad that you liked the articles. And thanks for sharing the link.