Data binding is an important part of any application.AngularJS provides several different methods of data binding.There are several data binding expressions which we can use depending on the scenario.
Some of the useful directives related to data binding are
ng-bind
This directive is useful if we want to perform one way data binding from the source to the target but not the other way.So the changes in the source are reflected in target but the changes in the target are not reflected in the source
we use this directive as:
<div ng-bind="Property"/>
We will define the following controller for the examples here
var app = angular.module('app', []); app.controller('Ctrl',['$scope', function ($scope) { $scope.Name="AngularJS"; }]);
We apply the above controller to the body element.In the div we set the data binding using the ng-bind directive.
<body ng-app="app" ng-controller="Ctrl"> <div ng-bind="Name"/> </body>
There is another easy to understand syntax for ng-bind directive ,data binding expression .Instead of applying ng-bind directive to an element we can use the following
{{Property}}
So we can write the above example using expression as
<body ng-app="app" ng-controller="Ctrl"> {{Name}} </body>
Using any of the syntax for data binding is similar and when rendered both displays the text “AngularJS”.
ng-model
If we want to use two way data binding in AngularJS application then we can use the ng-model directive.We can apply the ng-model directive to any input element
such as text.The effect of applying this directive is that when the value in the input element changes the value in the viewmodel also changes.The value in the input element can change as a result of user entering a new value.
We can use ng-model directive as:
<input type="text" ng-model="Property"/>
So we can use this directive using the Name property defined in our controller as:
<input type="text" ng-model="Name"/>
The effect of applying this directive is that the Name value in $scope changes.Using this directive the changes are automatically propagated in both ways from target to source and vice versa.
If we use the following markup which one-way binds the Name property in the $scope to the div.Also we are binding the text input to the same Name property using ng-model.
<body ng-app="app" ng-controller="Ctrl"> <div>Hello {{Name}}</div> <input type="text" ng-model="Name"/> </body> </html>
The effect is that as we enter the text in the text box it is displayed on the screen.
ng-non-bindable
Sometimes we may require to use the brackets as a literal rather than being interpreted as a data binding expression.In such a scenario we can use the ng-non-bindable directive.
For example the following directive will render the text Hello {{Name}}
<div ng-non-bindable> Hello {{Name}} </div>
If we had not used this attribute then the value of the Name.
ng-repeat
This directive is used to display items in a collection of items.So if the collection contains 5 items then the specified HTML template will be rendered for the 5 items in the collection.
If we define the employees collection as:
var app = angular.module('app', []); app.controller('Ctrl',['$scope', function ($scope) { $scope.Name="AngularJS"; $scope.employees= [ {Name:'Mark',Department:'HR',Id:1}, {Name:'Tim',Department:'Finance',Id:2}, {Name:'Frank',Department:'Administration',Id:3} ]; }] }]);
We can render the items in the employees collection as:
<body ng-app="app" ng-controller="Ctrl"> <div ng-repeat="emp in employees"> Name:{{emp.Name}} Department:{{emp.Department}} Id:{{emp.Id}} </div> </body>
When using ng-repeat directive HTML template is rendered for the items in the collection .We are not required to write custom code to loop through all the items in the employees collection and rendering the template for each item.
So the data binding directives in AngularJS simplifies many common data binding scenarios without the need to write custom logic.

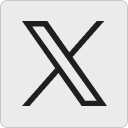
Leave a Reply