There are lots of third party logging frameworks available for implementing logging functionality in a .net application.Some of the popular logging frameworks used with .net applications are:
- log4net
- NLog
- ELMAH
.NET Core provides support for logging API in the Microsoft.Extensions.Logging namespace which is useful for capturing logs.It could be used as a facade which integrates with different logging frameworks.The advantage is that API remains consistent.Different logging frameworks have providers for the .NET Core Logging API.This means that you just need to call the required extension method.
So you can use the in built .net core framework for implementing logging functionality in .net core applications or integrate it with other frameworks such as NLog or Serilog.Currently .NET Core Logging API supports the following providers:
- Console
- Debug
- EventSource
- EventLog
If you need a provider which is not currently included in the API you have the option to integrate with other logging framework.It is as simple as calling an extension method for the required logging provider.
For consuming the .net core logging framework you need to add the Microsoft.Extensions.Logging namespace to your application.You can use it to your controller class if you want to implement logging in a controller:
using Microsoft.Extensions.Logging;
Code language: CSS (css)
Declare variable of type ILogger in the class as:
private readonly ILogger _logger;
Code language: PHP (php)
ILogger is the main interface for implementing logging functionality.It is defined in the Microsoft.Extensions.Logging namespace.Some other interfaces which you you might need when implementing logging are:
- ILoggingProvider
- ILogger
- ILoggerFactory
ASP.NET Core has built in support for dependency injection.To implement logging in your asp.net core controller you need to pass a reference of ILogger as a parameter in your controller constructor:
public HomeController(ILogger<HomeController> logger)
{
}
Code language: HTML, XML (xml)
inside the constructor assign the ILogger parameter to the _logger variable you have declared above:
public HomeController(ILogger logger)
{
_logger = logger;
}
Code language: PHP (php)
Now you can write log in any action method as:
_logger.LogInformation("Writing test log");
Code language: JavaScript (javascript)
Here we have used constructor injection for injecting the ILogger interface in the controller.You can also inject the ILoggerFactory in the constructor:
private readonly ILogger _logger;
public HomeController(ILoggerFactory logger)
{
_logger = logger.CreateLogger("HomeController");
}
Code language: PHP (php)
The .net core framework comes with some default providers.You can use other logging providers as well for implementing the logging functionality.To add a new logging provider you need to add the required providers in the BuildWebHost method of the Program class .You need to pass the method in the ConfigureLogging method in Program class as:
WebHost.CreateDefaultBuilder(args)
.UseStartup()
.ConfigureLogging(logging =>
{
logging.AddConsole();
})
.Build();
Code language: JavaScript (javascript)

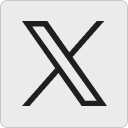
Leave a Reply