IHttpActionResult is an interface which was introduced in WebAPI 2.It is used to create HttpResponseMessage which is one of the return types in WebAPI.
Use of IHttpActionResult
Using IHttpActionResult to return HttpResponseMessage object instead of directly returning the HttpResponseMessage object has the following advantages:
Since we return an interface from the action method ,it makes it easier to unit test our action methods.
It promotes separation of concerns since the logic to create the HttpResponseMessage object is moved to different class.This class implements the IHttpActionResult interface.
public class HTMLResponse : IHttpActionResult { string _html; HttpRequestMessage _requestMessage; public HTMLResponse(string html, HttpRequestMessage requestMessage) { _html = value; _requestMessage = requestMessage; } public Task<HttpResponseMessage> ExecuteAsync(CancellationToken cancellationToken) { var response = new HttpResponseMessage() { Content = new StringContent(_html), RequestMessage = _requestMessage }; return Task.FromResult(response); } }
We can use the above HTMLResponse as:
public IHttpActionResult Get() { return new HTMLResponse ("HTML Response", Request); }
So as you can see in the above Get method ,it just creates an instance of HTMLResponse and returns it.This makes the Get method cleaner since the responsibility of creating HTMLResponse object is moved to separate class.
System.Web.Http.Results namespace
System.Web.Http.Results namespace contains different implementations of the IHttpActionResult interface.For example JsonResult<T> is a generic class which is used to return JSON data and status code of OK.
ApiController class defines several helper methods which can be used to create implementations of IHttpActionResult interface.
For example the following will return the generic Json<T> object.
public IHttpActionResult Get() { obj=studentRespository.Get(id); return Json<Student>(obj); }

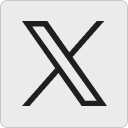
Leave a Reply