Media Type or MIME type specifies the data being transfered between the client and server in HTTP request or response.
The MIME type for image is image/png.So if the MIME type is specified as image/png for Content-Type of the response then the client will know that the response is an image and will render it appropriately.
There are many other MIME types such as:
- text/html
- image/png
- application/xml
Once the type of request or response is determined WebAPI needs to serialize or deserialize the data. WebAPI uses media formatter to serialize or deserialize the data.
For example if action method returns an object as:
public Students Get() { return studentRespository.Get(); }
As the students data needs to be send in response body so serialization of students data is required.This serialization is
performed by the MediaTypeFoarmatter.Two of the inbuilt MediaTypeFoarmatters are:
- JsonMediaTypeFormatter For performing JSON serialization
- XmlMediaTypeFormatter For performing XML serialization
The base class for all Formatters is MediaTypeFormatter.If we want to create a new media type formatter then we need to create a new class which derives fromĀ MediaTypeFormatter or BufferedMediaTypeFormatter.BufferedMediaTypeFormatter dervies from MediaTypeFormatter class.
These classes are defined in the System.Net.Http.Formatting namespace in System.Net.Http.Formatting.dll assembly.
So to create a new media type formatter we can declare a class as:
public class CustomFormatter : MediaTypeFormatter { }
then in the constructor we add the media type as:
public class CustomFormatter : MediaTypeFormatter { public CustomFormatter() { // Add the supported media type. SupportedMediaTypes.Add(new MediaTypeHeaderValue("application/javascript")); } }
Override the CanWriteType and CanReadType methods
To specify that Formatter supports serialization
public override bool CanWriteType(System.Type type) { return true; }
To specify that Formatter supports deserialization
public override bool CanReadType(Type type) { return true; }
Override the WriteToStream and ReadFromStream methods.
public override void WriteToStream(Type type, object value, Stream writeStream, HttpContent content) { using (var writer = new StreamWriter(writeStream)) { Serialize(value,writeStream); } }
Similarly to perform deserialization override ReadFromStream method.

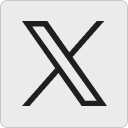
I couldn’t find any working example that showed or taught how to create .png or .jpeg media Formats and upload any image files in the MVC WebApi2 Environment by AJAX. Can you help me with this?