Binary Search is one of the methods of searching an item in a list of items.Here we will look into how to implement binary search in C#.
We first need to calculate the middle element in the list and then compare the element we are searching with this middle element.
To calculate middle element we use the formula:
middle element = highest element + lowest element / 2
After we calculate the middle element we compare the element we are searching for with this element.There can be three conditions:
- if value is equal to the middle element
- if value is less than the middle element
- if value is greater than the middle element
class BinarySearch
{
public static int Find(int[] numList, int val)
{
int low = 0;
int high = numList.Length-1;
int mid = 0;
while (low < =high)
{
Console.WriteLine(string.Format("low=0,high={1}",low,high));
mid = high +low/ 2;
if (val == numList[mid])
{
Console.WriteLine(string.Format("found value"));
return mid;
}
if (val > numList[mid])
{
Console.WriteLine(string.Format("value is less than the middle element"));
low = mid + 1;
Console.WriteLine(string.Format("low=0,high={1},mid={2}", low, high, mid));
}
else
{
Console.WriteLine(string.Format("value is greater than the middle element"));
high = mid - 1;
}
}
return -1;
}
}
public static void Main()
{
var location = BinarySearch.Find(new int[] { 1, 2, 3, 4,5,6,7,8,9 }, 6);
Console.WriteLine(location);
}

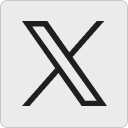