Angular 2 applications are designed for better application organization.Two of the main concepts related to organization of code in Angular 2 are components and modules.Both Components and modules are created by using the decorator functions.NgModule in Angular 2 is used to create a module.
Here we will see how to implement a simple module in Angular 2.
To create Angular 2 module we use the decorator function @NgModule().To this function we pass metadata information in the form of a metadata object.
Following are the main steps to implement a simple module in Angular 2 application
1.Import the NgModule which is required to create a new module
import { NgModule } from '@angular/core';
2. Declare a new class.This is the class which will represent our module.
export class AppModule{ }
We have named our class as AppModule since it represents the root module in our application.
3.Decorate this class with the @NgModule() decorator function,
@NgModule({ }) export class AppModule{ }
4.Add the metadata in the @NgModule().Here we are adding bootstrap.This metadata represents the bootstrap component.
@NgModule({ bootstrap: [ AppComponent ] })
If required we can include import declarations in the @NgModule decorator function as:
@NgModule({ bootstrap: [ AppComponent ], imports: [ // put all your modules here BrowserModule, // Angular 2 out of the box modules TranslateModule, // some third party modules / libraries ] })
The above decorator specifies AppComponent in the bootstrap property.What this means is that there is a component called AppComponent which will launch our application.
There is AppComponent in App in app.module.ts file.
In the app.module.ts we launch the application by calling the bootstrapModule() function.
//app.module.ts platformBrowserDynamic().bootstrapModule(AppModule);

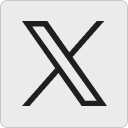