AngularJS makes common data binding tasks easy to implement.We set the viewmodel for our application using the $scope in the controller and access it in the view.AngularJS automatically updates the view when the value of the $scope changes.
One common feature is iterating over a collection of items.We can implement this scenario easily using the ng-repeat directive.The ng-repeat directive creates HTML template for each item in a collection of items.
So instead of manipulating the HTML using JavaScript we just specify what template we require for an item in a collection.For example if we have a collection of items defined as:
var app = angular.module('app', []); app.controller('Ctrl',['$scope', function ($scope) { $scope.employees=[ {Name:'Mark',Department:'HR',Id:1}, {Name:'Tim',Department:'Finance',Id:2}, {Name:'Frank',Department:'Administration',Id:3} ]; }]);
Then we can render the above collection using ng-repeat.Following will display Name and Department properties for every employee in a div:
<div ng-repeat="emp in e.employee"> <i>Name</i>{{emp.Name}} <i>Department</i> {{emp.Department}} <i>Id</i> {{emp.Id}} </div>
ng-repeat-start and ng-repeat-end directives in AngularJS
ng-repeat-start and ng-repeat-end directives in AngularJS are used to display groups of data.These are used to display HTML markup for a group of items.
The above employees array has only three elements but a real application may consist of thousands of records.Also an organization may have lots of departments.With ng-repeat we will not be able to group the records based on a certain criteria such as Department.
To group the data in a collection on a certain property angular 1.2 and above versions provides the ng-repeat-start and ng-repeat-end directives.Using these directives we can easily group the data in a collection.
So building on the above example but this time with more records we define the employees array as:
$scope.employees=[ {department:'Finance' ,employee:[ {Name:'Mark',Department:'HR',Id:1}, {Name:'Tim',Department:'Finance',Id:2}, {Name:'Frank',Department:'Administration',Id:3} ] } , {department:'HR' ,employee:[ {"Name":"John", "Id":4}, {"Name":"Sam", "Id":5}, {"Name":"Ravi","Id":6} ] } , {department:'Administration' ,employee:[ {"Name":"Ashish", "Id":7}, {"Name":"Peter", "Id":8}, {"Name":"Craig","Id":9} ] } ]
We can group the above items in the employees collection by using the ng-repeat-start and ng-repeat-end directives as:
<table ng-app="app" ng-controller="Ctrl"> <tr ng-repeat-start="e in employees"> <td> <b> {{e.department}} </b></td> <td> </tr> <tr ng-repeat="emp in e.employee"> <td > Name{{emp.Name}} Id {{emp.Id}} </td> </tr> <tr ng-repeat-end> <td> {{e.Name}} </td> </tr> </table>
Above markup renders the list of employees in a table grouped by Department name

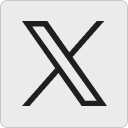