Switch statement is one of the conditional statements in c# such as if else.Switch statement in C# is a concise way of writing conditions specially when your code is cluttered with lots of else if conditions,which is making code difficult to read.
It is used for selecting one of the blocks from a list of code blocks.The code block to execute is determined based on the value of the match expression.The keywords used in switch statement are:
- switch It accesses the matchExpression in the beginning.
- case It defines various constant values which are matched with the matchExpression
its syntax is
switch(matchExpression) { case Value1: code block1; break; case Value2: case Value3: code block2; break; default: code block default; break; }
Following is a simple switch case statement:
switch(num) { case 1: Console.WriteLine("Case 1"); break; case 2: Console.WriteLine("Case 2"); break; case 3: case 4: Console.WriteLine("Case 3"); break; default: Console.WriteLine("not found"); ; break; }
It will check the value of the num variable in the beginning and based on that value it will execute the appropriate case statement.If none of the case statement matches then default case will be executed and “not found” will be displayed.
You can notice above that we used same code block for both Case 3 and Case 4.This is allowed in C# and you could write common logic for multiple cases this way.

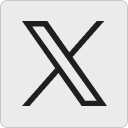