Three types in System namespace are used for working with date and time values.People wrongly believe that DateTime is a class,all of these are structs.
Date time structs for working with date and time values:
- DateTime Stores value as ticks and DateTimeKind enum for identifying date and time.
- DateTimeOffset Stores value as timestamp (called offset) from UTC.
- TimeSpan Represents interval of time
DateTime struct
DateTime is the most commonly used type for working with date time values.It defines the following properties.All of these are get only,you can not modify after you create a DateTime value:
- Date
- Day
- Hour
- Minute
- Month
- Year
- Second
Creating DateTime object
Using constructors
You pass the values for day,month,year,hour,minute and second.Some of the constructors it provides are:
DateTime(int year, int month, int day); DateTime(int year, int month, int day, int hour, int minute, int second); public DateTime(int year, int month, int day, int hour, int minute, int second, int millisecond); public DateTime(int year, int month, int day, int hour, int minute, int second, int millisecond, DateTimeKind kind); public DateTime(int year, int month, int day, int hour, int minute, int second, int millisecond, Calendar calendar);
For example to create a date object for the year 2019,day 1 and month 9:
var dateObj = new DateTime(2019, 9, 1);
After you have created a datetime variable you can access its various properties,to get the appropriate value.
Adding DateTime values
To add datetime values you use the AddXXX methods.
At a minimum you need to specify at least day,month and year or tick.You specify day,month and year as int
Some of the common methods are:
AddDays(double value); AddHours(double value); AddMinutes(double value); AddMilliseconds(double value); AddMonths(int months); AddYears(int value);
Examples
Adding days
You can add one day to a DateTime object as:
var dateObj = new DateTime(2019, 9, 1); var newdateObj = dateObj.AddDays(1);
Substracting Days
Outputting DateTime
You can use ToString() method on any date time object to print its value.By default it prints the short datetime value.
var newdateObj = new DateTime(2019, 9, 2); Console.WriteLine(newdateObj.ToString());
Output:
9/2/2019 12:00:00 AM
TimeSpan
You use TimeSpan to find the difference between two date time values.For example you can find the difference between the following beginning and stopDate as:
DateTime beginingDateTime = DateTime.Now; DateTime stopDateTime = DateTime.Now.AddDays(1); TimeSpan difference = stopDateTime - beginingDateTime;

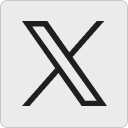