Some commonly asked Core Java interview questions.
What are the main concepts of OOPS?
Following are the important Oops concepts:
- Encapsulation hiding internal implementation details.Clients can access the object only through the public interface such as methods.
- Abstraction Once you implement encapsulation by hiding internal implementation details of the object you implement abstraction.Abstraction means defining public interface to access the object.
- Polymorphism Means same form different behavior. In the context of Object oriented programming it means the same function can behave differently depending on the
passed arguments. - Inheritence a new class can reuse the functionality of an existing class by deriving from an existing class.The new class acquires the methods and properties of the existing class.This is an example of is-a relationship.
What are different types of polymorphism in Java?
There are two types of polymorphism
- Runtime polymorphism Method overriding.Method to invoke is decided at runtime.
- compile time polymorphism Method overloading.Method to invoke is decided at compile time.
What is JIT compiler?
Compilation in Java happens in two phases:
- Java code to Bytecode
- Bytecode to machine code of CPU
JIT compiler compiles Bytecode to machine code at runtime.Since this compilation happens at runtime ,it is known as JIT(Just in time compilation).It is through Bytecode that Java is platform independent.Bytecode is not specific to any specific CPU so it can execute on different platforms.
What is JVM?
JVM is Java virtual machine.JVM converts the Bytecode to machine code.There is a separate JVM for every platform.
What is Constructor?
Constructor is like a normal method but has some differences from a normal method.Instead of invoking explicitly ,constructor is automatically called when an object of the class is created.It’s not required to define constructor in our class.If we don’t define a constructor then default constructor is created by Java.It initializes the variables with default values.A constructor is not inherited by the derived class.
How inheritence is implemented in Java?
inheritence is implemented in Java using the extends keyword.We define a subclass which extends a superclass as:
class sublcass extends superclass { //class members,e.g methods and fields }
class which extends the parent class is called subclass while parent class is called super class.
What is generalization in Java?
generalization means extracting common attributes from two or more classes into a common super class.generalization in Java is implemented using inheritence.
For example common traits from employees of different departments such as employee number and company name can be extracted into a common super class.Different employee classes can then inherit from this employee super class.
Any behavior which can invoked on an object of super class can also be invoked on an object of child class.
What is association?
In association relationship classes depends upon each other but each class is independent.Each class can be instantiated independently.
What is an Interface?
Interface is just a contract which consists of method signatures.Interface doesn’t consist of implementation details. Implemetation is provided by the classes which implements the interface.Interface methods can be only public and abstract.The variables can be public,static or final.So Interface can consist of variables but just constants.
An interface is a description of a set of methods that conforming implementing classes must have.
Which class is the superclass of all the classes?
java.lang.Object is the super class of all the java classes
Does Java support multiple class inheritance?
Java does not support multiple class inheritance.A class in Java can inherit only from single class.
Which method is called first in Java application?
The main() method is called first in a Java application.Its signature is:
public static void main(String args[])
Can a single Java file define multiple classes?
A Java source file can contain multiple classes.But only single class in the source file can be public.
What is garbage collection?
Garbage collection is used to free the unused memory. By removing memory no longer being used by object references ,memory is made available for the program.

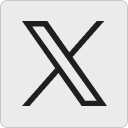