Attributes provide information about html elements.They are specified as name value pairs in the opening tag of the html element.
<element attribute=”value”/>
e.g
<input type=”text”/>
When a web page is rendered the attribute values are read and the page is rendered accordingly.So to update a web page dynamically attributes are important.
For example when a user hovers over a textbox ,for styling the textbox attribute values can be set.To update page dynamically attr() method is used.Not only the attr() method is useful for setting the values of the matched elements it is also useful for fetching the attribute values of the elements.
Fetching attribute values
In the following example we have a text field .To fetch the contents of the textbox attr() method can be used as:
var text=$("input").attr("value");
Id:<input type="text" value="1" />
The value of the variable text above will be 1.
In the following example we have multiple text fields.
Id:<input id="id" type="text" value="1" /> Product:<input id="product" type="text" value="Computer" /> Price:<input id="price" type="text" value="10000" />
To access the value of each of these text fields we use the $(“input”).each() function:
$(document).ready( function () { $("input").each(function() { alert( $(this).attr("value")); } ) });
If we don’t use the each() function then value attribute of only the first input element is retrieved.
Setting Attribute values
The same attr() method is also used for setting the attribute value.Apart from the attribute name ,a attribute value is also passed to the attr() method.
If we pass a single parameter to the attr() method then the method returns the attribute value.If two parameters are passed to the attr() method then the attribute value is set.
Here we are using the sample example.But instead of explicitly setting the values in html ,we are setting the values using attr() method.
Id:<input id="id" type="text" value="1" /> Product:<input id="product" type="text" value="Computer" /> Price:<input id="price" type="text" value="10000" /> $(document).ready( function () { $("#id").attr("value",1); $("#product").attr("value", "Computer"); $("#price").attr("value", "10000"); });
The result is same as explicitly setting the values in html.

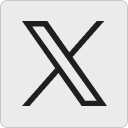