Following are some of the most common PHP developer interview questions and answers.
What is PHP?
PHP is a server side scripting language used for developing web applications.It is an object oriented programming language .
How do you embed PHP scripts in a webpage?
You can embed PHP scripts in a web page by enclosing the PHP script within <?php and ?>.Following a simple PHP script:
<?php echo "Hello PHP"; ?>
What is the use of “echo” in php?
echo is used to display text in the page.It is used as
<?php echo 'string value'; ?>
How do you declare variables in PHP?
Variables are identifier with a “$” prefix.As PHP is a loosely typed language ,types are not specified when declaring variables unlike other languages such as Java.For example you can declare a variable called name as:
$name='PHP'
What are local and global variables?
- Local variable is declared inside a function and can be used inside that function.
- Global variable is declared outside any function and can only be used outside any function.
Can you access global variable from inside a function?
You can access a global variable from inside a function by using the $GLOBALS super global variable.It allows to access global variables even from within functions.
How do you declare a function in PHP?
You declare a function in PHP using the function keyword followed by the function name.To declare a function called message:
function message() { echo "Hello PHP"; }
How do you declare array in PHP?
You can declare an array in PHP as:
- Declare a variable like a normal variable.Identifier name should begin with the $ prefix.
- Assign the array to the variable by using the array function.
Following is an example of array:
$empIds=arrays(1,2,3,4);
How do you get the length of the array?
You use the count() function to get the number of elements in an array.
How can you concatenate two strings?
You can concatenate two strings by using the dot “.” operator as:
$str1 . $str2;
This will concatenate the strings str1 and str2.

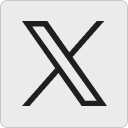