Packages and Modules are used for organizing python application.Packages are represented as directories and modules are represented as single files.To use a module you need to import it first.
Before writing your first Python program you need to download Python 3 as we will be using Python 3 in these tutorials.After installing Python you need to set up environment on your computer.
You can use any text editor to write Python program.Sublime text can also be used for writing Python programs.
To create a new module in python follow the below steps
1.create a directory
create a python file having the extension .py.You can name the file anything just make sure it has the extension .py
Copy the following contents to the directory
def helloWorld(): print('Hello from Python')
2.navigate to the above directory using the command prompt
type “python” to start the python interpreter
import the module using the following command
import python_hello_world
instead of python_hello_world you need to use the name of the python file which you have created in step 1.
3.Call the function which you have defined in the above module
python_hello_world.helloWorld()
In this simple program we have used print() function.This is aa inbuilt function in Python.It just prints the string on screen.
To exit the Python interpreter on windows just press Ctrl+Z.

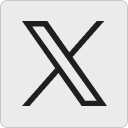