ArraySegment is a generic collection implemented as a structure. ArraySegment is used to create a collection based on an array.We can use ArraySegment in C# if we are using the framework version 4.7.2 .
So if you have defined an array as:
int[] nums = { 1, 2, 3, 4, 5 };
then you need to define arraysegment based on the above array as below.please note that we are passing integer type as arraysegment type parameter.This is because arraysegment is based on integer array.
ArraySegment<int> arrObj = new ArraySegment<int>(nums);
So the following is not valid:
ArraySegment<string> arrObj = new ArraySegment<string>(nums);
In this example we are defining an arraysegment for the entire array.If we want we can also define arraysegment for a portion of the array instead of entire array.
For example the following will print the items 3 and 4 as we starting from index 2 and including 2 elements.
int[] nums = { 1, 2, 3, 4, 5 }; ArraySegment<int> arrObj = new ArraySegment<int>(nums,2,2); foreach(var item in arrObj) { Console.WriteLine("item ={0}", item); }
So if you need perform different operations on different parts of the array then you can use ArraySegment struct.
Array property
If you require to access the original array wrapped by the array segment then you can use the Array property.For example if you want to refer to the array referred to by the arrObj then you can use the Array property as:
arrObj.Array
Offset property
If you need to find the difference between the first element in the array segment from the initial element in the original array then you can use the Offset property.For example if you want to find the Offset of the arrObj then you use:
arrObj.Offset

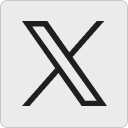
Leave a Reply