LINQ extension methods are used to perform queries on the lists and collections.We can use C# for the LINQ queries rather using any other language such as SQL.
We can Compare List elements in C# using LINQ ,by using the extension methods.
To compare lists using LINQ there is a SequenceEqual extension method provided by the framework.If we have two arrays arr1 and arr2 declared as:
string[] arr1 = { "A", "B", "C" }; string[] arr2 = { "A", "B", "C" };
then we can compare the above arrays using the SequenceEqual() method as:
bool result = arr3.SequenceEqual(arr2);
The result variable will contain the value true as arr1 and arr2 contains the same elements.
Instead of arrays we can compare two lists using the SequenceEqual() method.So if there are two lists of strings declared as:
List<string> arr4 = new List<string>(){ "A", "B", "C" }; List<string> arr5 = new List<string>() { "A", "B", "C" };
then we can compare them as:
bool result = arr4.SequenceEqual(arr5);
result will still have the value true since the two lists are identical.Above we are comparing two generic lists of strings.
But there is an important point to consider:
When using SequenceEqual to compare two lists or arrays ,the items in the two collections should be the same in the same ordinal.For example if the first list contains the value “A” in the first position then the second list should also contain the value “A” in the first position.
Though to compare List elements in C# using LINQ the framework provides the SequenceEqual method.This method compares elements based on the ordinal.We can implement logic to compare elements independent of the ordinals.
So if we now have two arrays with the same values but in different positions then SequenceEqual will return the value false.
string[] arr2 = { "A", "B", "C" }; string[] arr3 = { "B", "C", "A"}; bool result = arr2.SequenceEqual(arr3);
The result of the above comparison will return false.
So as evident we need to use some different approach to compare the List elements in C# using LINQ.
To compare collections which may contain same values but in different positions we can use custom logic.Here we will use the LINQ extension methods for comparing the two collections.
If two collections contains the same elements then the following two points will be true:
- The size of two collections will be same
- Each collection will contain the element of the other collection
We can implement logic to verify these using LINQ as:
private static bool ComapreLists(string[] arr2, string[] arr3) { bool _areEqual = false; if (arr3.Length == arr2.Length) { var filteredSequence = arr3.Where(x => arr2.Contains(x)); if (filteredSequence.Count() == arr3.Length) { _areEqual = true; } } return _areEqual; }
Here we are checking if arr2 contains every element of arr3 and if the size of two collections is same.We can call the above method as:
string[] arr2 = { "A", "B", "C" }; string[] arr3 = { "B", "C", "A"}; bool result=ComapreLists(arr2, arr3);
CompareLists method above will return true if the two passed array arguments contains the same elements.
So we can easily implement the method to Compare List elements in C# using LINQ.If we don’t use LINQ then our logic will be more complicated.

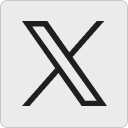
Leave a Reply