The classes required for the LINQ to XML functionality are part of the System.Xml.Linq namespace.So if we want to implement LINQ to XML then we need to use the classes defined
in the System.Xml.Linq namespace.
Some of the important classes required to create an XML document are:
- XElement It represents an element in the XML.
- XDocument Represents XML document
- XAtrribute It represents an attribute of an element
Generating XML using XDocument
As XDocument class represents an XML document so we can create an object of this class if need to create XML document.This object represents an in-memory XML document on which we can perform queries.
XDocument xmlDoc = new XDocument(new XElement("root", new XElement("element1", "content1"), new XElement("element2", "content2") ) );
XDocument defines several overloaded constructors.One of the constructors accepts an array of objects.We pass a object of XElement which defines the root and nested elements.The above XDocuments object represents the following XML.
<root> <element1>content1</element1> <element2>content2</element2> </root>
We can use Console.WriteLine(xmlDoc) to write it to the console.
Generating XML using XElement
XElement is one of the important classes when we need to create XML using LINQ to XML.It provides constructors which we can use to create a hieracrchy of elements.
Creating a single root element having string content
XElement xmltree = new XElement("Root", "root");
Creating a root element containing a single child element
XElement xmlDoc = new XElement("Root", new XElement("Employee","employee1"));
Creating a root element having child elements which further have child elements
XElement xmlDoc = new XElement("Employees", new XElement("employee", new XElement("name", "ashish")), new XElement("employee", new XElement("name", "amit"))
The above code generates the following markup
<Employees> <employee> <name>ashish</name> </employee> <employee> <name>amit</name> </employee> </Employees>
The constructor of XElement is overloaded.One of the constructors is defined as:
XElement(XName name,params object[] content)
In the second parameter we can pass another XElement object.This allows us to create a hierarchy of nested elements.

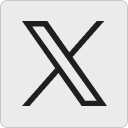
Leave a Reply