DbContext is the main class that acts as an interface between the domain classes in our application and the database.To interact with the database we use the DbContext class.
We work with the entities in our application ,adding ,updating or deleting entities as required.DbContext is responsible for populating the entities from the database and for updating the database from the entities.
In the prior versions of Entity Framework, ObjectContext was used instead of DbContext. DbContext is a wrapper around the ObjectContext class.DbContext is easier to use than the ObjectContext for performing different operations.
Whenever we update entities in our application ,EF tracks the changes to those entities.Once we are done updating the entities we just inform the EF to save our changes in the database.The main class we use for performing these operations on the entities is the DbContext class.
Some of the important members defined by this class are:
Member Type | Name | Use |
Constructor | DbContext() | Creates a new instance.The database name to which connection will be made is determined from class name of the DbContext derived class. |
Constructor | DbContext(DbConnection, Boolean) | Creates a new instance using an existing database connection. |
Constructor | DbContext(String) | Creates a new instance using the connection string passed as an argument. |
Property | ChangeTracker | Returns the change tracker for the DbContext |
Property | Configuration | Allows us to configure the DbContext |
Method | SaveChanges() | Saves all changes made using this context to the underlying database. |
Method | Set<TEntity>() | Returns the DbSet<T> where T is the type of Entity for which DbSet is required. |
Method | OnModelCreating() | We can override this method to manage the relationships between the entities. |
In the below example we are declaring UserContext class ,which is derived from the DbContext class.In the UserContext class we have declared a property of type Users.This Users property represents the Users table in the database.
public partial class UserContext : DbContext { public UserContext() { } public virtual DbSet<User> Users{ get; set; } } public class User { public string Name{get;set;} public int Id { get; set; } public string Password { get; set; } }
We usually declare a context class derived from the DbContext context when we use the code first approach in Entity Framework.On executing the above code a database will be created automatically if it doesn’t exist.

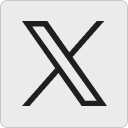
Leave a Reply