Expression bodied function members is a new feature introduced in C# 6.It makes methods more concise ,easy to read and understand.
Before C#6 we used to write the methods having a method body surrounded by curly braces.Whether we are returning a single value or not our method consists of multiple lines of code. There was no other way but to write the method body.
Now with the Expression bodied function members in C# 6 we can write the function members using syntax similar to lambda expressions.
Expression bodied members combine the syntax of function members and the lambda expressions.For example we write an expression bodied method as a combination of lambda expression and the method signature.So methods doesn’t contain the body surrounded by the curly braces { and } and instead consists of just the lambda arrow and the expression.
method signature => expression
For example if we want to write a simple method which calculates the sum of the parameters below is the only option to write such methods.
public int Add(int a,int b) { int sum = a + b; return sum; }
Now using expression bodied function members in C# 6 we can write the above method using more concise syntax
public int Add(int a, int b) => a + b;
Expression bodied members allows Function member’s to define their body in a very concise way.
We can use expression bodies not only with methods but also with other function members such as properties.For example if we have the following property
public int Year { get { return DateTime.Today.Year; } }
we can write it as:
public int Year => DateTime.Today.Year;
Following are some of the important things about Expression bodied function members in C# 6
- We can not declare body with curly braces for an expression bodied function member.
- While declaring properties by using an expression bodied function member we can declare only read only properties.
- They are just another syntax of writing the function members such as methods and properties and doesn’t improve the performance.
- Similar to normal function members expression bodied members can be declared with different accessibility modifiers such as public ,protected.
- They are different from lambda expressions though syntax resembles lambda expressions.

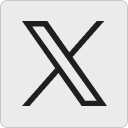
Leave a Reply