We can create XML without using LINQ to XML by directly using the XmlDocument and the related classes.
When we create XML using this approach we are manipulating the XML document.It is also not very easy to understand the XML manipulation logic.We can not tell by just looking at the code the XML tree being created.
For example for creating the following XML tree
<Employees> <Employee> <EmpNumber>1</EmpNumber> <Name>Mike</Name> </Employee> </Employees>
we use the following logic
XmlDocument doc = new XmlDocument(); XmlElement employees = doc.CreateElement("Employees"); XmlElement employee = doc.CreateElement("Employee"); XmlElement name = doc.CreateElement("Name"); name.InnerText = "Mike"; XmlElement empNumber = doc.CreateElement("empNumber"); empNumber.InnerText = "1"; employee.AppendChild(employee); employees.AppendChild(empNumber); employees.AppendChild(name); doc.AppendChild(employees);
Though it creates the above XML tree correctly but just by looking at the above code we can not relate it to the XML tree.
By using LINQ to XML we can create the same XML tree in a single statement.Following LINQ to XML statement will create the same XML tree as above:
XElement xmlDoc = new XElement("Employees", new XElement("Employee", new XElement("Name", "Mike"), new XElement("EmpNumber", "1")));
This is possible because of the functional construction provided by LINQ to XML.
To understand how we can create XML in a single statement its important to understand the XElement class.XElement defines several overloaded constructors which simplifies creation of XML trees.
Constructor | Use | Example |
public XElement(XName name) | Creates XElement object having a specific name | XElement el = new XElement(“Employee”); |
public XElement(XName name,object content) | Creates XElement object with a specific name and content |
Using text content: mainElement = new XElement(“Employees”, “Employee Text”); using XElement: mainElement = new XElement(“Employees”,new XElement(“Employee”, “First Employee”)); |
public XElement(XName name,params object[] content) | Creates XElement object with a specific name and a group of content | new XElement(“Employees”, new XElement(“Employee1”), new XElement(“Employee2”), new XElement(“Employee3”), new XElement(“Employee4”)); |
If we look at the above code marked in bold then we can see that one of the overloaded constructors of XElement expects content as the second argument.We can pass either text content or another XElement object here.It is by passing objects of XElement that we are able to create XML tree using the functional construction.

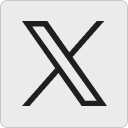
Leave a Reply