Lazy object initialization is useful for class members which are not always required by the consumers of the class.For example suppose a class member variable accesses an external resource such as web service.Since this variable is accessing an external resource hence creation of the class could be expensive.
In the following example Employee class defines a property EmployeeDetails.The EmployeeDetails property fetches its value using a web service.
class Employee { public string Name{get;set;} public string Address { get; set; } public int Salary { get; set; } } class Organization { private string[] departments; public string[] Departments { get { departments = new string[4]; departments[0] = "HR"; departments[0] = "Finance"; departments[0] = "IT"; departments[0] = "Admin"; return departments; } string[] EmployeeNames { get; set; } private Employee[] employeeDetails; Employee[] EmployeeDetails { get { //fetch details from web service method return employeeDetails; } set; } }
So if we create an object of Organization type and don’t reference EmployeeDetails field even then EmployeeDetails object will be created and populated.
Following code will populate EmployeeDetails object even though it is not referenced anywhere.
Organization org = new Organization(); var departments=org.Departments;
EmployeeDetails is an array of Employee type.The value of EmployeeDetails is fetched from the web service.Creating a proxy object and fetching the details of employees can take some time especially if there are thousands of records.In such a case it can be time consuming to create an object of Organization.
If the EmployeeDetails variable is never used then populating EmployeeDetails is not necessary.In such scenarios we can implement lazy initialization for the variable.Instead of providing custom implementation there is generic class provided by the framework.
Lazy<T> is a generic class in the System namespace .It can work with different datatypes.An object of Lazy<T> type is not created until it is actually referenced by the consumer.
So we can declare an EmployeeDetails variable as:
class Organization { private string[] departments; public string[] Departments { get { departments = new string[4]; departments[0] = "HR"; departments[0] = "Finance"; departments[0] = "IT"; departments[0] = "Admin"; return departments; } string[] EmployeeNames { get; set; } private Lazy<string[]> employeeDetails; public Lazy<string[]> EmployeeDetails { get { //fetch details from web service method return employeeDetails; } set; } }
Now the EmployeeDetails object will only be created if it is referenced by the consumer.
Organization org = new Organization(); var departments=org.Departments; var employeeDetails = org.EmployeeDetails.Value;
Value is a readonly property which returns the underlying data.
Lazy object initialization in C# is used when a class refers a type which is expensive or time consuming to create.Instead of immediately creating an object of the referenced type it is deferred till it is actually referenced.In such scenarios it can be useful to save both time and resources required for creating a referenced type.

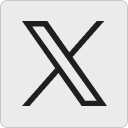
Leave a Reply