Any variable of type DateTime can be assigned appropriate values for handling date and time data.Any value which lies between the DateTime.MaxValue and DateTime.MinValue can be assigned to a DateTime variable.
Following example demonstrates this.
Ticks is a property of DateTime variable which represents the variable as an integer.In the following example we are validating if a variable lies within the valid range for a datetime variable.
long largeNumberofTicks = long.MaxValue; //largeNumberofTicks = 9223372036854775807 bool isValidValue; // DateTime.MaxValue.Ticks=3155378975999999999 // DateTime.MinValue.Ticks=0 if (largeNumberofTicks >= DateTime.MinValue.Ticks && largeNumberofTicks <= DateTime.MaxValue.Ticks) { isValidValue = true; } else { isValidValue = false; }
In the above example isValidValue will be set to false as long.MaxValue>DateTime.MaxValue.Ticks.
DateTime Min value
DateTime.MinValue is the minimum value that can be assigned to a DateTime variable.It is equal to “1/1/0001 12:00:00 AM”.
DateTime Default value
As value types can not be assigned null values like reference types so they always have some default initial value.
DateTime is also a value type ,so it can not be assigned a null value.So DateTime variable always have some value.
By default DateTime variable is assigned DateTime.MinValue value.
So if we want to check if a datetime variable has been assigned a value we can use the following code
if (dateVariable == DateTime.MinValue) { //minimum value }
We have declared Person class below
class Person { public string Name { get; set; } public DateTime dateOfBirth; }
In the following code we are creating an object of person class and checking the value of dateOfBirth.
class Program { static void Main(string[] args) { Person person = new Person(); if (person.dateOfBirth == DateTime.MinValue) { Console.WriteLine("dateOfBirth has a value of " + DateTime.MinValue); } } }
On executing the above code we get the following output
dateOfBirth has a value of 01-01-0001 12:00:00 AM
DateTime Max Value
DateTime.MaxValue just like DateTime.MinValue is a readonly field.It represents the maximum value that can be represented by a datetime variable.The value of this field is 23:59:59.9999999 UTC, December 31, 9999
if(dt.Ticks> DateTime.MaxValue.Ticks) { //invalid date } else { //valid date }

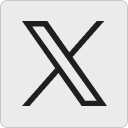
Leave a Reply