One of the most common exceptions while developing a C# application is a null reference exception.I believe most of us have encountered this exception at some point of time.The reason for this exception is that we are trying to access a type member when that type reference is null or not assigned an object.
For example if we consider the following example
public static void Main(string[] args) { Employee emp = new Employee(); emp.Name = "Mike"; Console.WriteLine("Employee Name{0} Employee City", emp.Name, emp.Details.City); } class Employee { public string Name { get; set; } public DetailInfo Details { get; set; } } public class DetailInfo { public int PostCode { get; set; } public string City { get; set; } public string State { get; set; } }
In the above example Employee class contains a reference to DetailInfo class.If we execute the above code then we will get null reference exception.Since Details property is null so we get this exception.
In the prior versions of C# we can prevent the above exception by checking the null value in the if condition as
if(emp.Details!=null) Console.WriteLine("Employee Name{0} Employee City", emp.Name, emp.Details.City);
Though the above null condition will check for the null value of the string parameter we can still get the null reference exception.If need to display the employee details in uppercase then we will get the null reference excetion
if (emp.Details!=null) Console.WriteLine("Employee Name{0} Employee City", emp.Name.ToUpper(), emp.Details.City.ToUpper());
To prevent the exception we will have to put one more null check as
if (emp.Details != null) { if(emp.Details.City!=null) Console.WriteLine("Employee Name{0} Employee City", emp.Name.ToUpper(), emp.Details.City.ToUpper()); else Console.WriteLine("Employee Name{0} Employee City", emp.Name.ToUpper()); }
And if we want to display other Employee details then we will have to increase that many null checks.
So from the above example it is clear that null checks are commonly used to prevent exceptions because of null references.
Null-conditional Operator
C# 6 includes the null conditional operator.
It verifies if the object instance for null reference before accessing the members of the object.If obj is an object of class A and Name is a property defined by class A then we can use this operator as:
obj?.Name
If obj is null then Name is not accessed and null reference exception is not thrown.
Above is a very simple use of this operator.It is more useful in the case of classes which contains references to other classes.
Using the null conditional operator in C# 6 we can rewrite the above code to display the Employee details as
Console.WriteLine("Employee Name{0} Employee City", emp?.Name.ToUpper(), emp?.Details?.City?.ToUpper());
So as we can see in the above example by using the Null Conditional Operator of C# 6.0 we have replaced multiple if conditions with this operator.It is more useful in the case of nested references as in the above example.

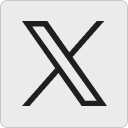
Leave a Reply