Optional parameters and named arguments were added to C# in version 4.0.They are an important feature of the language which makes working with methods easier in many scenarios.
Named arguments
Often we need to call methods which have lot of parameters.So if a method has 10 parameters and many of them are of the same data type then it is really cumbersome to understand which
argument would map to which method parameter.This is where named arguments are useful.
For example we have a method defined as:
public static void Calculate(int a,int b,int c,int d,string e,int f,double g,int h ) { //method body }
if we try to call the above method then it can be challenging to identify which argument will map to which parameter.
Calculate(1, 44,346, 8, "hello", 433, 1.0, 67);
Though we are calling the Calculate method using the correct arguments but if look at the above method call it is not easy to understand the use of each argument.We can not say just looking at the above method call to which parameter value 67 will map to.
Instead of matching the arguments to the method parameters using the position as above we can
use the named arguments which maps the method argument to the parameter using the name
of the parameter.
Below we are calling the same method using the same arguments but this time we are using
the named arguments instead of positional arguments
Calculate(d:8, c:44,a:1,e:"hello",f:433,h:67,g:1.0,b:44);
The above method call is much easier to understand as we are easily able to identify which arguments will map to which method parameters.For example we can easily say that value 67 will be assigned to the method parameter “h”.
Optional parameters
Many times when we create a method we want some default parameter value to be used if
the caller of the method doesn’t provide any valid value for a method parameter.In such
a scenario instead of letting the caller of the method provide any dummy value for the
parameter we can let the caller of the method omit the value for such a parameter.
For example without using the optional parameters the caller of the UpdateDetails method is required to pass null value or empty string for the designation parameter.As the caller don’t know what value to pass for the designation parameter he is forced to pass null or it is empty string.
If the value of the designation parameter is null or empty string then a default value is assigned to the designation parameter.
public void UpdateDetails(string name, string designation, string address) { if (string.IsNullOrEmpty(designation)) { designation = "Application developer"; } //method body }
Instead of assigning the value of the designation parameter based on its value passed by the caller we can just assign a default value to the designation parameter
public static void UpdateDetails(string name, string address, string designation = "application developer") { //method body }
Now the caller of the method is not required to pass empty or null value to designation parameter. Caller can just call the UpdateDetails method without passing any value for the designation parameter.
UpdateDetails("Mark", "France");
The important thing to understand while using the optional parameters is that once we declare a method parameter as optional the parameters declared in the parameter list after the optional parameter should also be optional.
For example the following is invalid and compiler will not compile the below code
public static void UpdateDetails(string name,string designation = "application developer", string address) { //method body }
Optional parameters and named arguments in C# 4.0 are useful to easily implement some common scenarios.
Named arguments can be useful for scenarios which otherwise requires method overloading.For example we can replace the following overloaded methods
public int Add(int a, int b) { return a + b; } public int Add(int a, int b, int c) { return a + b + c; }
with the below method using optional parameters
public int Add(int a, int b, int c=0) { return a + b + c; }
So optional parameters in C# 4.0 are useful for avoiding redundant code.Instead of defining lots of method overloads we can define just a single method with default parameter values.

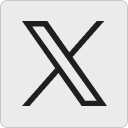
Leave a Reply