Path is location of a file or a directory.This location can be on a disk , can be a network location or can refer to the location on some device.The location can be relative to some other location in which case it is called the relative path.If the location is not specific to any other location then it is called an absolute path.
For example if we have following directory structure
C:Current DirectoryFilesFile.txt
then if the current location is C:Current Directory ,the relative path to File.txt will be FilesFile.txt
and the absolute path will be C:Current DirectoryFilesFile.txt
To work with the paths in .NET System.IO.Path class is provided.Though we can work with paths without using this class ,Path makes it convenient and reduces chances of using an incorrect path.
Path is a static class in the System.IO namespace which contains some useful static methods and properties for working with the paths.It is important to understand that when we use these methods of the Path class the actual file or directory is not modified.We can retrieve or manipulate the string representation of the path but the actual path remains unchanged.
Also if we are passing some file or directory path to these methods we should verify if the directory or file actually exists.Since the path is not verified by these methods so we will not be informed if we pass some invalid or non-existent path to these methods.
Some of the useful methods related to working with Files in the Path class are:
Path.GetFileName(path)
This method returns the file name including the extension from path which is passed as an argument to this method
We can call this method as
string path= @" "C:Filesfile.txt"; Console.WriteLine("File Name={0}", Path.GetFileName(path)); //the above will display the output "file.txt"
Path.GetFileNameWithoutExtension(path)
This method returns the file name without the extension from the path which is passed as an argument to this method
string path= @" "C:Filesfile.txt""; Console.WriteLine("File Name={0}", Path.GetFileNameWithoutExtension(path)); //returns "file"
Path.GetPathRoot(path)
Returns the root directory in the specified path.For example in the path “C:Filesfile.txt” C: is the root directory.
string path = @"C:Filesfile.txt"; Console.WriteLine("path={0}", Path.GetPathRoot(path)); //it will display the string C:
Since we specified the absolute path in the C: drive we get the root directory as C:.If we specify the absolute path without specifying the directory name then we will get the root directory as “” which means the current directory.
If we call this method by passing the argument @”Filesfile.txt” then we will get the
root directory as “” which is current directory.
Path.GetTempPath()
Returns the path to the temporary folder on the users machine.This method doesn’t take any arguments.
Temp folder is located in the Users folder.So calling this method returns a path similar to:
C:UsersUserNameAppDataLocalTemp
Path.Combine(path1,path2)
Combines two fragments of a path to form a single combined path.Instead of concatenating the paths we can use this method.
Console.WriteLine(Path.Combine("C:", @"Filesfile.txt"));//C:Filesfile.txt Console.WriteLine(Path.Combine(@"C:", @"Filesfile.txt"));//C:Filesfile.txt Console.WriteLine(Path.Combine(@"C:Files", @"file.txt"));//file.txt
Path class in C# for working with file paths reduces chances of errors of forming an incorrect path ,since it checks if path separators are valid.
If we concatenate two strings representing paths and if the combined path is invalid then we are not informed about it.For example if join the two paths as:
string combinedPath = @"C:" + @"Filesfile.txt";
then the value of combinedPath will be C:\Filesfile.txt which is invalid path.
But if we join the above two paths using the Combine method then we will get the following valid path.
Filesfile.txt
The Combine method is smart enough not to concatenate the two “” separators together.

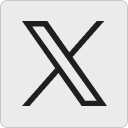
Leave a Reply