A common requirement while developing any application is connecting to a database and for connecting to the database connection details such as database name and server location are required.We provide these details using the connection string.Instead of specifying these details every time we need to connect to the database we keep the connection string in the configuration files such as web.config or app.config.
We define the connection string in the connectionStrings element as:
<connectionStrings> <add name="SqlConnection" connectionString="Data Source=.;Initial Catalog=SampleDB;Integrated Security=True"/> </connectionStrings>
Reading connection strings in c#
To read the values in our code we need to use the static ConfigurationManager class.To use this class we need to add a reference to the System.configuration namespace
To read the connection string we need to use the ConnectionStrings property of the ConfigurationManager class.
var connString = ConfigurationManager.ConnectionStrings["SqlConnection"].ConnectionString;
If we run the above code the connection string defined in the configuration file will be assigned to the connString variable
Now we can use this connection string to create SqlConnection object by passing this string in the constructor as:
SqlConnection conn = new SqlConnection(connectionString)
In the versions of .NET prior to 3.5 we can read the connection string using the following code:
string connStr = ConfigurationSettings.AppSettings["connectionString"];
Please note using ConfigurationSettings class to read the connection strings is deprecated.In the versions 3.5 and above ConfigurationManager should be used instead to read the connection strings.
ConfigurationManager is a sealed class which is used for reading the configuration information and can be used to read different configuration data.It provides different methods for reading different configuration elements from the various types of configuration files.
Similar to the ConnectionStrings ConfigurationManager provides the AppSettings property for reading the AppSettingsSection data in the configuration file.

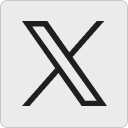
Leave a Reply