StreamWriter class is part of the System.IO namespace.It is used for writing to streams in particular encoding.We can use it to write text files.
Write to the text file
To write to a text file using StreamWriter we implement the following steps
Create an instance of StreamWriter.We pass the name of the file we want to create or modify in the constructor
Below we are passing the string Test.txt to the StreamWriter constructor.This will create a file Test.txt if it doesn’t exist.
StreamWriter sw=new StreamWriter("Test.txt");
If we want to append to an already existing file then we use another constructor which accepts a boolean value.
Below will create a StreamWriter object which will append to the file Test.txt if it already exists.
StreamWriter sw=new StreamWriter("Test.txt",true);
Following will overwrite the Test.txt if it exists.Existing contents of the file are replaced with the new contents.
StreamWriter sw=new StreamWriter("Test.txt",false);
To write to the file there are different method which we can use
Write(String) write the string passed as the argument WriteAsync(String) writes the string asynchronously Write(String, Object) writes formatted string
There are different write methods provided for writing different data types such as Single,Double,
Write(Double) Write(Single) Write(Boolean) Write(Object)
For writing asynchronously to the text file we use WriteAsync method.
Following will write to the file Test.txt.
using(StreamWriter sw=new StreamWriter("C:\\Test.txt",true)) { sw.WriteAsync("Test Line 1"); sw.WriteAsync("Test Line 2"); }
Reading from text file
To read from the text file we use StreamReader class.
For reading the text file we implement the following steps
Create an instance of StreamReader.We pass the name of the file we want to read in the constructor
StreamReader sr = new StreamReader("C:\\Test.txt")
Call the ReadLine() method of the StreamReader object until all the lines have been read
Following will read all the lines in the file Text.txt
using (StreamReader sr = new StreamReader("C:\\Test.txt")) { string line; while ((line = sr.ReadLine()) != null) { Console.WriteLine(line); } }

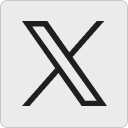
Leave a Reply