ReadOnlyCollection is a collection of items that can not be changed. To understand the use of
ReadOnlyCollection first lets see the behaviour of ordinary collections in .NET
We declare a List<T> of strings in C# as
List<string> namesLst = new List<string>();
now if we want to prevent users from modifying this list we may try to use the readonly modifier.As readonly is used to prevent changing the value of a variable once it is declared so this modifier seems appropriate for our use.
So we may declare the List<string> as
static readonly List<string> namesLst = new List<string>();
Now if we try to add the items to the above collection as
namesLst.Add("Mark"); namesLst.Add("Mike"); namesLst.Add("John"); namesLst.Add("Jenny");
Even though we have declared the collection as readonly we are able to add the items to the collection using the above code.
So readonly modifier though appropriate for declaring readonly variables doesn’t makes the collection as readonly.To make the collection as immutable which can not be modified we need to wrap our collection in ReadOnlyCollection instance as:
ReadOnlyCollection<string> namesReadOnlyLst; namesReadOnlyLst = new ReadOnlyCollection<string>(namesLst);
If we expose the ReadOnlyCollection then it becomes immutable and items can not be added to this collection.
If we have an array we can make it as readonly by using the AsReadOnly() method of the Array class.We use this method as:
Array.AsReadOnly(array)
For example if we have an array of names we can make it as immutable as:
ReadOnlyCollection<string> namesReadOnlyLst; string[] names = new string[] { "Mark", "Mike", "John", "Mark", "Jenny" }; namesReadOnlyLst = Array.AsReadOnly(names);
Now there is no way an item can be added to the namesReadOnlyLst collection above.As ReadOnlyCollection doesn’t contains Add method or an indexer to add items to the collection so we can not add new items to a ReadOnlyCollection.
Example of ReadOnlyCollection
In the following SavingsAccount class we have a property Users which is of type ReadOnlyCollection<User>.Since it is ReadOnlyCollection so clients of the SavingsAccount class will not be able to add new users to the collection.
For adding a new user we have to use the AddUser(User user) method which is declared as private.Since we have declared the method as private hence we can add a new user from only within the SavingsAccount class.
class SavingsAccount { private List<User> _users = new List<User>(); public ReadOnlyCollection<User> Users { get { return _users.AsReadOnly(); } } private void AddUser(User user) { _users.Add(user); } }
So for adding new users to the collection can call the AddUser(User user) method from within the class.For example we can load the users from the database in the constructor.This ensures that the sensitive will not be tempered with by the consumers of the class.
So as ReadOnlyCollection in .NET prevents a collection from being modified ,we can expose our collection as ReadOnlyCollection if we want to ensure that it can not be modified by the users of our class.

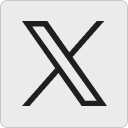
Leave a Reply