We pass data to the method using arguments.When we call the method we pass arguments which are assigned to the parameters defined by the method.After executing the logic method can return values to the caller using the return statement.
For example the following method accepts two parameters and returns the sum of the parameters as an integer return type:
public int Add(int x,int y) { return x + y; }
When we pass values to the method using method arguments ,the parameters are assigned the arguments used to call the method.Now if the method modifies the parameters then the arguments used to call the method are not effected.This is useful since it prevents the side effects of modifying the parameter values from propagating to the rest of the program.So we can say that arguments are passed by value by default as the copy of the arguments is assigned to the parameter.
But sometimes it may be required that the argument and parameter are same or argument and parameter refer to the same memory location.We can easily implement this using the ref and out parameters.
ref parameters
When we pass the ref type parameters the parameter becomes an alias for the argument.So whatever changes we make to the method parameter affects the argument used to call the method.
public static void UpdateValues(ref int x, ref int y) { x = x + 10; y = y + 10; } static void Main(string[] args) { int a=1, b=1; UpdateValues(ref a, ref b); Console.WriteLine("a={0},b={1}", a, b); }
If we execute the above code then we can see that the values of x and y are updated and is reflected even outside the method:
The values of x and y are reflected in the calling method since the variables a and b refers to the same memory location as x and y.This happens when we pass the parameters by reference.
out parameters
c# requires that every method parameter is assigned a value when the method is called.For example if we define a method as:
public static void UpdateValues( int x, int y) { x = x + 10; y = y + 10; }
then if we try to call the above method without assigning the parameter values then the compiler will complain that the argument variables are unassigned
But what if don’t have the parameter value when calling the method or if we want to return multiple values from the method which are not known when the method is called ? As we can not use normal method parameters ,we use out parameters in such as a scenario.
static void Main(string[] args) { int a, b; UpdateValues(out a, out b); } public static void UpdateValues( out int x, out int y) { x = 0; y = 0; x = x + 10; y = y + 10; }
In the above code though we have not assigned the parameters still the compiler doesn’t complain as we have declared the parameters as out parameters.
ref and out parameters in c# are used for passing the parameters by reference instead of passing the parameters by value.out parameters differs from the ref parameters as we can pass the parameters without assigning them when calling the method.

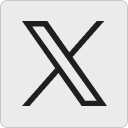
Leave a Reply