LINQ consists of a set of query operators.These query operators transforms a sequence .Sequence is just a collection of objects.Sequences in LINQ are used by the LINQ operators in queries.
There are two sequences which can be used in LINQ queries
- IEnumerable
- IQueryable
LINQ operators can be applied to any collection which implements any of these interfaces.There are lots of collections which implements these types.Some of the common collections which implements these types are
- Array
- Generic list ,List<T>
- LinkedList<T>
- HashSet<T>
- Collection<T>
- Dictionary<TKey,TValue>
The query operators are defined in the System.Linq namespace and are defined as extension methods.So to apply these operators we need to import the System.Linq namespace.
In the following example we are querying an array which contains different weather conditions.We are selecting all the weather conditions which are not equal to “Hot”
string[] weather = { "Hot", "Cool", "Moderate" }; var result=weather.Where(x => x != "Hot").Select(x=>x.ToString()); foreach(var item in result) Console.WriteLine(item);
The collection weather will contain the values “Cool” and “Moderate”
There are few important things to understand about the above code.Where is a query operator which filters the items in a collection based on a condition
- Select is a query operator which is used for projecting the items in the result collection
- Select returns an object of type IEnumerable<TResult> where TResult represents the type of items in the result collection
So in the above example instead of the following line
var result=weather.Where(x => x != "Hot").Select(x=>x.ToString());
we can use the below
IEnumerable<string> result = weather.Where(x => x != "Hot").Select(x => x.ToString());
IQueryable<T>
Another Sequence in LINQ is IQueryable<T>.Like IEnumerable<T> it is used in LINQ queries. IQueryable<T> implements the IEnumerable<T> interface.
The difference is that IQueryable<T> is used to execute queries remotely.It is used with query providers such as LINQ to SQL.Different query providers can provide their own implementations of IQueryable<T>.
IQueryable<T> constructs queries using expression tree unlike IEnumerable<T> which constructs queries using delegates.Expression tree is used to create an actual query on the remote data source.
AsEnumerable()
The AsEnumerable() method can be called on collections which implements IEnumerable<T>.For example DataTable type in ADO.NET has its own implementation of query operator.By calling AsEnumerable() method on the table we can use the Standard query operators.If we don’t use AsEnumerable() on the DataTable then the custom implementation of the DataTable is used instead.
DataTable table = new DataTable(); table.AsEnumerable().Select(x => x);

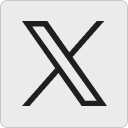
Leave a Reply