When working with collections sometimes we need to perform certain operations only for some of the elements in a collection and skip the rest of the elements.Skip operator is used in such cases.
Skip operator in LINQ skips n number of elements in a collection of elements.It returns the remaining elements after skipping the n elements in a collection
It is used as:
Collection.Skip(n)
where Collection is an object of type IEnumerable<T>
n can be any integer value
The following example will return all the elements except the first element which is 1.
List<int> numbers = new List<int> {1,2,3,4,5,6,7,8}; var result = numbers.Skip(1);
The Skip() operator is useful when used together with the OrderBy() operator.The elements in a collection can be ordered either ascendingly or descendingly and then the topmost or lowermost elements can be returned by using the Skip() operator.
In the following example we have a list of students.Each student has a name and marks which he has scored.
We can use the Skip() operator along with the OrderBy() operator to return the top 3 students based on his or her score.
List<Student> students = new List<Student> { new Student{Name="Robin",Marks=88} ,new Student{ Name="John",Marks=56 } ,new Student{ Name="Tom",Marks= 66} ,new Student{ Name="Janet",Marks= 76} ,new Student{ Name="Punit",Marks= 77} }; var studentSelected = students.OrderBy(x=>x.Marks).Skip(3);
SkipWhile
SkipWhile operator skips the elements in a collection until a certain condition returns true.Once the condition returns false the remaining elements are returned.
If we want to find the students scoring more than 60 marks we can use the SkipWhile operator as:
var studentSelected = students.OrderBy(x=>x.Marks).SkipWhile(x=> x.Marks < 60);
Skip and SkipWhile skips the elements depending on the ordinal or a condition.But once an element is returned by these operator the remaining elements are also returned.So for example if we remove the OrberBy operator then once a student is found having more than 60 marks then the remaining students are also returned irrespective of the marks scored.
var studentSelected = students.SkipWhile(x=> x.Marks < 60);
This will return all the elements since Robin is at the beginning of the list and he is having more than 60 marks.

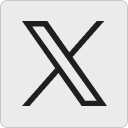
Leave a Reply