One of the new features of C# 6.0 is the “Using Static” statement.In the previous versions we can import only the namespaces with the help of using statement.Now we can import Static classes also.For example to import the namespace for Linq we write
using System.Linq;
and to import the namespace for generic collections we write
using System.Collections.Generic;
But the important thing is that we can only import the namespaces with the using statement.For example we can not import the System.Console class.If we write the following statement
using System.Console;
then we get the following compiler error
‘using namespace’ directive can only be applied to namespaces; ‘Console’ is a type not a namespace.
But in C# 6 we can import the Static class members as well.For example we can use the following using statement to import the static class members of the Console class
using static System.Console;
The advantage of importing the static class members is that we do not have to explicitly qualify each and every member of the static class.For example if we want to use the WriteLine method of the Console class then we need to fully qualify the WriteLine method as:
Console.WriteLine("Hello C# 6!");
But in C# 6 we can just call the static method without qualifying it with the class name.So instead of the above method call we can call the WriteLine method as:
WriteLine("Hello!");
Though for single statements it may not appear to be so useful ,but in scenarios where lots of statements are calling the Static methods this can be more useful.For example if we are using many WriteLine static method calls then our code may look as:
Console.WriteLine("Name: {0}",name); Console.WriteLine("Details: {0}",details); Console.WriteLine("Address: {0}",address); Console.ReadLine();
The above Console statements are redundant and serve no purpose then qualifying the WriteLine and ReadLine static methods.
We can replace the above code with the following in C# 6.0:
WriteLine("Name: {0}",name); WriteLine("Details: {0}",details); WriteLine("Address: {0}",address); ReadLine();
In the above code we have imported the Console class members with the using static Statement in C#6.0.This makes the code much easier to understand because the redundant Console class name has been removed.

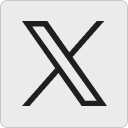
Leave a Reply