Arrays are useful for storing a collection of data.For example if we have a group of student data related to students in a School then we can define an array as:
class Student { public int Id; public String Name; public String Address; } String[] students=new String[10000]; students=getStudents();
The method getStudents() may fetch the list of students from webservice or database.
So arrays are useful for storing a set of data.But the main problem with arrays is that the size of an array is fixed and it can not be changed later on.For example if the number of Students in school increases then we can not increase the size of the array after we have declared the above array.
One solution is to declare the arrays with suffixiet size to accomodate the future requirments.So in the above case we can declare the array of size 15000 ,since we assume the number of students can not be more than that.
String[] students=new String[15000];
But the problem now is that we have declared the size of the array which is much more than the actual number of Students.This means that extra memory is bing allocated for Students.Though that memory will never be used.
For these types of scenarios Arraylist is a better solution.Arraylist differs from arrays since the size of an Arraylist can be altered at runtime.So if we don’t know how many elements an array will contain we can use an ArrayList.
Following are the steps to declare and add elements to an ArrayList
1. Import the required namespaces.
ArrayList class implements the List interface.It extends the AbstractList class.To work with ArrayList<T> in our code we need to import the following package from java.util library:
import java.util.ArrayList;
2. Create an ArrayList object
Once we have imported the required package we can create an object of the generic Arraylist as:
//create a list of Students
public class Student { public int Id; public String Name; public String Address; } ArrayList<Student> students = new ArrayList<Student>();
3. Add elements to an ArrayList
For adding elements to arraylist we use the add() method
//add item to list Student student=new Student(); student.Id=1; student.Name="John"; student.Address="Autralia"; students.add(student);
For adding element at a specific index we use an overload of add() method as:
student=new Student(); student.Id=2; student.Name="Amit"; student.Address="India"; students.add(1,student);
Note that we have provided the index as the first parameter.
4. Reset elements at a specific location
We can reset element at a specific index using the set() method as:
student=new Student(); student.Id=2; student.Name="Marc"; student.Address="UK"; students.set(1,student);
In the above code we are replacing the Student at second position ,”Amit” with Student “Marc”
5.Retrieve elements from the arraylist.
We can retrieve an item from the arraylist using the get() method as:
student=students.get(1);
6.Get the number of elements in ArrayList
There is a size() method which returns the number of elements in an ArrayList at any given time.
So if we execute the above code in sequence then size() will return the value 2.
System.out.println(students.size());
output: 2

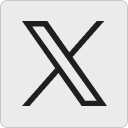
Leave a Reply