Many times we need to declare multiple variables of the same type to hold related values.For example list of students in a class.As there are lot of students in a class so to store the names of students in variables we can declare the variables as:
String Student1="John"; String Student2="Priya"; String Student3="Amit"; ...
So using variables to store students names means we need to declare lot of variables.This approach has the following disadvantages:
- In case of big collection of data ,the number of variables can increase.
- It is difficult to manage lot of variables specially when assigning or fetching these values.
- The code is difficult to read and understand.
Because of disadvantages arrays are prefered way to store large collection of related data.
For example if we have to assign and read the list of students in a class using variables we will do it as:
InputStreamReader in=new InputStreamReader(System.in); BufferedReader br=new BufferedReader(in); String Student1=br.readLine(); String Student2=br.readLine(); ... System.out.println(Student1); System.out.println(Student2);
As you can see there are 2 problems in this approach.
- We have to know in advance the exact number of variables we are going to assign
- Assigning and reading the values makes the code difficult to understand
We can easily assign and read values using array as:
String[] student=new String[2]; InputStreamReader in=new InputStreamReader(System.in); BufferedReader br=new BufferedReader(in); for(int count=0;count<student.length;count++) { student[count]=br.readLine(); } for(int count=0;count<student.length;count++) { System.out.println(student[count]); }
Arrays can be assigned and retrieved using a for loop.This makes arrays easier to work with a group of data unlike ordinary variables.

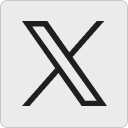
Leave a Reply