Enum is a group of numeric contants.TypeScript supports enum since version 0.9.
Enums are useful for representing values which can have one of a fixed set of values.For example we can represent
employee type with the following enum:
enum EmployeeType{ Permanent = 1, Contractor, }
One important thing to understand is the type of values enum members can be assigned.Enum members can be assigned values which can be computed at compile time.In the above example both the enum members are assigned constant values.
Enum member Permanent is assigned value 1 explicitly.The value of enum member Contractor is computed from the value of Permanent enum member.The value of Contractor is 2 ,which is calculated by adding 1 to the previous enum member value.
Though we have assigned contant value in the above example ,but we can assign any numeric expression.The only condition is that the numeric expression should be computed at compile time.
We can declare enum variable of the as:
let employeeType = EmployeeType.Permanent;
Converting Enum value to string
For converting string value of an enum member use the following:
EmployeeType[EmployeeType.Permanent]
The above code will return the value of the enum member Permanent of enum EmployeeType to string.

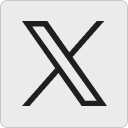
Leave a Reply